Asynchronous JavaScript And XML (Ajax) is the cornerstone of communication between your client-side and server-side code. Regardless of whether you use JavaScript, jQuery, Angular, React or any other client-side language, they all use Ajax under the hood to send and receive data from a web server. Using Ajax you can read data from, or send data to, a web server all without reloading the current web page. In other words, you can manipulate the DOM and the data for the web page without having to perform a post-back to the web server that hosts the web page. Ajax gives you a huge speed benefit because there is less data going back and forth across the internet. Once you learn how to interact with Ajax, you will find the concepts apply to whatever front-end language you use. In this article you are going to learn about the global Ajax events you can hook into.
Download Starting Projects
Instead of creating a front-end web server and a .NET Core Web API server in this article I have two sample projects you may download to get started quickly. If you are unfamiliar with building a front-end web server and a .NET Core Web API server, you can build them from scratch step-by-step in my three blog posts listed below.
- Create CRUD Web API in .NET Core
- Create .NET Core MVC Application for Ajax Communication
- Create Node Web Server for Ajax Communication
You can find all three of these blog posts at https://www.pdsa.com/blog. Instructions for getting the samples that you can start with are contained in each blog post. You are going to need blog post #1, then choose the appropriate web server you wish to use for serving web pages; either .NET MVC (#2) or NodeJS (#3).
Start Both Projects
After you have reviewed the blog posts and downloaded the appropriate sample projects to your hard drive, start both projects running. The first project to load is the Web API project. Open the WebAPI folder in VS Code and click on the Run | Start Debugging menus to load and run the .NET Web API project.
Open the AjaxSample folder in VS Code.
If you are using node, open the AjaxSample folder in VS Code, open a Terminal window and type npm install. Then type npm run dev to start the web server running and to have it display the index page in your browser.
If you are using the .NET MVC application, open the AjaxSample-NET folder in VS Code and click on the Run | Start Debugging menus to load and run the .NET MVC project. The index.cshtml page should now be displayed in your browser window.
Try it Out
Go to your browser for the front-end web server (localhost:3000) and you should see a page that looks like Figure 1. If your browser looks like this, everything is working for your front-end web server.
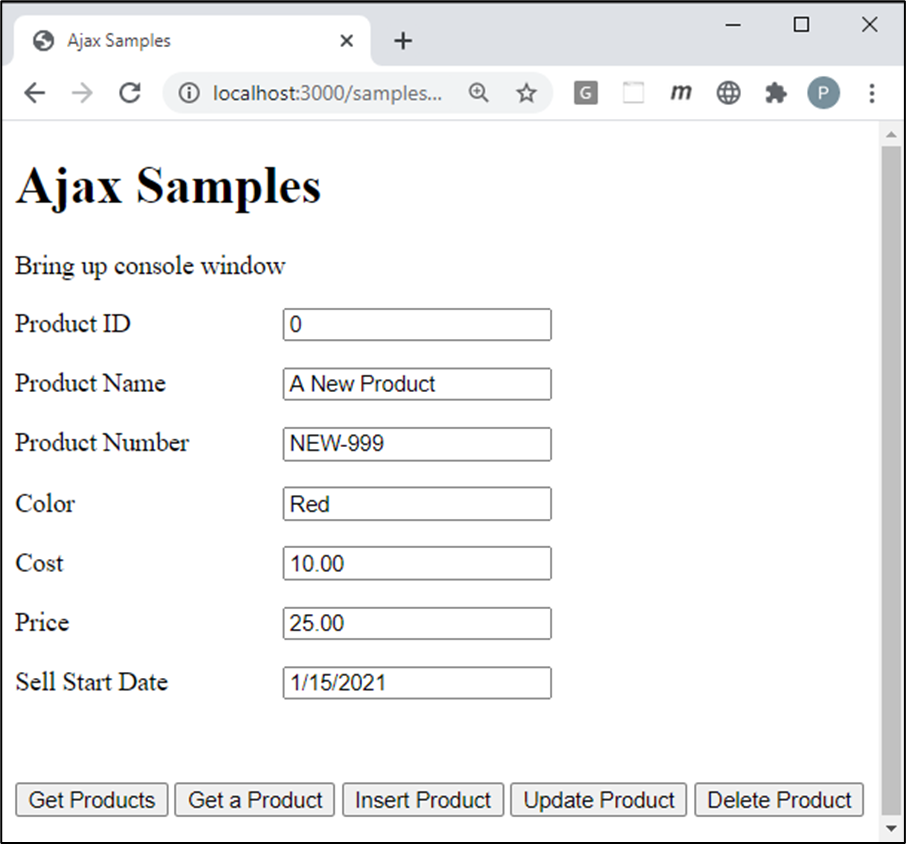
Open the Browser Tools in your browser, usually accomplished by clicking the F12 key. Click the Get Products button and you should see the product data retrieved from the Product table in the AdventureWorksLT database and displayed in your console window.
Install jQuery
If you have not already done so, you need to install jQuery into your node server project. If you are using the MVC application, jQuery is already installed, so you can skip to the next section of this article. Open the Terminal window in VS Code in your node server project and type the following command.
npm install jquery
After jQuery has been installed, open you index page and add a new <script> tag to use jQuery before all other <script> tags on the page.
<script src="/node_modules/jquery/dist/jquery.min.js"></script>
Get all Products
Open the index page and replace the get() function with the code shown in Listing 1.
function get() { $.ajax(URL) .done(function (data) { displayMessage("Products Retrieved"); console.log(data); }) .fail(function (error) { handleAjaxError(error); }) .always(function () { // Anything you want to happen here on either fail or done console.log("In the always() method"); }); }
Global Ajax Events
If you want to keep track of all Ajax calls made through your web application, there are several global events you may tap into as shown in Table 1.
Method | Description |
---|---|
ajaxComplete |
Called when an Ajax request is complete. |
ajaxError |
Called when an Ajax request has an error. |
ajaxSend |
Called just before an Ajax request is sent. |
ajaxStart |
Called when the first Ajax request starts. |
ajaxStop |
Called when all Ajax requests have completed. |
ajaxSuccess |
Called when an Ajax request completes successfully. |
The ajaxStart and ajaxStop Events
The ajaxStart() event is fired before you make the first Ajax call in your code. If you are sending multiple Ajax calls, this event only fires a single time. The ajaxStop() event fires after the last Ajax call has been made in your code. Open the index page and add the following two methods within the <script> tag on the page.
$(document).ajaxStart(function () { console.log("The first Ajax call is being fired"); }); $(document).ajaxStop(function () { console.log("The last Ajax call has completed"); });
Try it Out
Save the changes to the index page and go to the browser. Open the console window in your developer tools and click on the Get Products button. You should see the message from the ajaxStart() method, then the array of products retrieved, then the message from the .always() method, and finally the message from the ajaxStop() method.
The ajaxSend Event
The ajaxSend() event is fired before every Ajax call is made. There are two different forms of this event you can use, one with no arguments, and one with three arguments. You may use both in the same page. Open the index page and add the following lines of code within the <script> tag.
$(document).ajaxSend(function () { console.log("Ajax call is being sent"); }); $(document).ajaxSend(function (event, xhr, settings) { console.log(settings); });
NOTE: On thing that is possible is to add or modify settings prior to the call going out. For example, you could add something to the settings.url or maybe add a header to all calls going out.
$(document).ajaxSend(function (event, xhr, settings) { // Can add/modify settings if you want settings.url = "/resources/products.json"; console.log(settings); });
Try it Out
Save the changes to the index page and go to the browser. Open the console window in your developer tools and click on the Get Products button. You should see the message from the ajaxSend() event immediately after the message from the ajaxStart() event. Take a look at the settings object that is returned from the second form of the ajaxSend() event. There is a lot of useful property values in this object that help you identify which Ajax call fired this particular event.
The ajaxSuccess Event
Just like the ajaxSend() event the ajaxSuccess() event has two different ways you can respond to it. Add the following lines of code to the
$(document).ajaxSuccess(function () { console.log("An Ajax call has finished successfully"); }); $(document).ajaxSuccess(function (event, xhr, settings) { console.log(settings); });
Try it Out
Save the changes to the index page and go to the browser. Open the console window in your developer tools and click on the Get Products button. You should see the message from the ajaxSuccess() event immediately before the message from the ajaxEnd() event. The settings object is the same one you saw in the ajaxSend() event.
The ajaxComplete Event
The ajaxComplete() event fires after the ajaxSuccess() or the ajaxError() event. This is similar to the .always() method for a single Ajax call in that is always fires regardless of the success or failure of the call. The responseText property should have either the data returned or the error message from the last call.
$(document).ajaxComplete(function () { console.log("Ajax call is complete"); }); $(document).ajaxComplete(function (event, xhr, settings) { console.log(settings.url); console.log(xhr.responseText); });
Try it Out
Save the changes to the index page and go to the browser. Open the console window in your developer tools and click on the Get Products button. You should see the message from the ajaxComplete() event immediately after the message from the ajaxSuccess() event.
The ajaxError Event
If an ajax call encounters an error, the ajaxError() event is fired. There are two forms of this method, one that accepts no arguments, and one that has four arguments. The fourth argument is the actual error message that was thrown.
$(document).ajaxError(function () { console.log("Ajax call had an ERROR"); }); $(document).ajaxError(function (event, xhr, settings, thrownError) { console.log("Ajax call had an ERROR"); console.log(thrownError); console.log(settings); });
Try it Out
Go to your Web API project and open the ProductController.cs file. In the Get() method, add the following line of code just after the try statement.
[HttpGet] public IActionResult Get() { IActionResult ret = null; List<Product> list = new List<Product>(); try { throw new Exception("Test ERROR"); ... ... }
Save the changes to the ProductController.cs file and restart the Web API project.
Save the changes to your index page and go to the browser. Open the console window in your developer tools and click on the Get Products button. You should see the messages appear from the ajaxError() event along with the message from the thrownError argument.
Summary
While not something you are going to use all the time, the global events can help you log all the Ajax calls you make in your application. You might use the ajaxError() event to centralize where you log all of your Ajax application errors.
#jquery #ajax #javascript #pauldsheriff #development #programming